
C++ : A Beginner's Guide
2nd Edition
By:Â Herbert Schildt
Paperback | 1 December 2003 | Edition Number 2
At a Glance
576 Pages
Revised
23.5 x 19 x 3.5
Paperback
RRP $66.95
$36.25
46%OFF
About the Author
Herbert Schildt (Mahomet, IL) is a leading programming author. He is an authority on the C, C++, Java, and C# programming languages, and a master Windows programmer. His programming books have sold more than three million copies worldwide and have been translated into all major foreign languages. He is the author of numerous best-sellers including, C++: The Complete Reference, C: The Complete Reference, C++ from the Ground Up, Java 2: The Complete Reference, Java 2: A Beginner's Guide, C#: A Beginner's Guide, The Art of Java and many more. Schildt holds a master's degree in computer science from the University of Illinois.
Preface | p. xix |
C++ Fundamentals | p. 1 |
A Brief History of C++ | p. 2 |
C: The Beginning of the Modern Age of Programming | p. 2 |
The Need for C++ | p. 3 |
C++ Is Born | p. 4 |
The Evolution of C++ | p. 4 |
How C++ Relates to Java and C# | p. 5 |
Object-Oriented Programming | p. 7 |
Encapsulation | p. 7 |
Polymorphism | p. 8 |
Inheritance | p. 9 |
A First Simple Program | p. 10 |
Entering the Program | p. 11 |
Compiling the Program | p. 11 |
Run the Program | p. 12 |
The First Sample Program Line by Line | p. 12 |
Handling Syntax Errors | p. 15 |
A Second Simple Program | p. 16 |
Using an Operator | p. 17 |
Reading Input from the Keyboard | p. 19 |
Some Output Options | p. 21 |
Another Data Type | p. 22 |
Converting Feet to Meters | p. 24 |
Two Control Statements | p. 26 |
The if Statement | p. 27 |
The for Loop | p. 28 |
Using Blocks of Code | p. 30 |
Semicolons and Positioning | p. 32 |
Indentation Practices | p. 33 |
Generating a Table of Feet to Meter Conversions | p. 33 |
Introducing Functions | p. 36 |
The C++ Libraries | p. 38 |
The C++ Keywords | p. 38 |
Identifiers | p. 39 |
Module 1 Mastery Check | p. 40 |
Introducing Data Types and Operators | p. 43 |
Why Data Types Are Important | p. 44 |
The C++ Data Types | p. 44 |
Integers | p. 47 |
Characters | p. 48 |
Floating-Point Types | p. 50 |
The bool Type | p. 51 |
void | p. 53 |
Talking to Mars | p. 53 |
Literals | p. 55 |
Hexadecimal and Octal Literals | p. 56 |
String Literals | p. 56 |
Character Escape Sequences | p. 56 |
A Closer Look at Variables | p. 59 |
Initializing a Variable | p. 59 |
Dynamic Initialization | p. 60 |
Operators | p. 61 |
Arithmetic Operators | p. 61 |
Increment and Decrement | p. 62 |
Relational and Logical Operators | p. 64 |
Construct an XOR Logical Operation | p. 67 |
The Assignment Operator | p. 69 |
Compound Assignments | p. 70 |
Type Conversion in Assignments | p. 71 |
Expressions | p. 71 |
Type Conversion in Expressions | p. 72 |
Casts | p. 72 |
Spacing and Parentheses | p. 73 |
Compute the Regular Payments on a Loan | p. 74 |
Module 2 Mastery Check | p. 77 |
Program Control Statements | p. 79 |
The if Statement | p. 80 |
The Conditional Expression | p. 82 |
Nested ifs | p. 83 |
The if-else-if Ladder | p. 84 |
The switch Statement | p. 85 |
Nested switch Statements | p. 89 |
Start Building a C++ Help System | p. 91 |
The for Loop | p. 93 |
Some Variations on the for Loop | p. 95 |
Missing Pieces | p. 96 |
The Infinite for Loop | p. 97 |
Loops with No Body | p. 98 |
Declaring Loop Control Variables Inside the for Loop | p. 99 |
The while Loop | p. 100 |
The do-while Loop | p. 102 |
Improve the C++ Help System | p. 105 |
Using break to Exit a Loop | p. 108 |
Using continue | p. 110 |
Finish the C++ Help System | p. 111 |
Nested Loops | p. 115 |
Using the goto Statement | p. 116 |
Module 3 Mastery Check | p. 117 |
Arrays, Strings, and Pointers | p. 119 |
One-Dimensional Arrays | p. 120 |
No Bounds Checking | p. 123 |
Two-Dimensional Arrays | p. 124 |
Multidimensional Arrays | p. 126 |
Sorting an Array | p. 127 |
Strings | p. 129 |
String Fundamentals | p. 130 |
Reading a String from the Keyboard | p. 130 |
Some String Library Functions | p. 132 |
strcpy | p. 133 |
strcat | p. 133 |
strcmp | p. 133 |
strlen | p. 133 |
A String Function Example | p. 134 |
Using the Null Terminator | p. 135 |
Array Initialization | p. 136 |
Unsized Array Initializations | p. 140 |
Arrays of Strings | p. 141 |
Pointers | p. 143 |
What Are Pointers? | p. 143 |
The Pointer Operators | p. 144 |
The Base Type of a Pointer Is Important | p. 146 |
Assigning Values Through a Pointer | p. 147 |
Pointer Expressions | p. 148 |
Pointer Arithmetic | p. 148 |
Pointer Comparisons | p. 150 |
Pointers and Arrays | p. 151 |
Indexing a Pointer | p. 153 |
String Constants | p. 155 |
Reversing a String in Place | p. 156 |
Arrays of Pointers | p. 159 |
The Null Pointer Convention | p. 160 |
Multiple Indirection | p. 161 |
Module 4 Mastery Check | p. 163 |
Introducing Functions | p. 165 |
Function Fundamentals | p. 166 |
The General Form of a Function | p. 166 |
Creating a Function | p. 167 |
Using Arguments | p. 168 |
Using return | p. 170 |
Returning Values | p. 173 |
Using Functions in Expressions | p. 175 |
Scope Rules | p. 176 |
Local Scope | p. 177 |
Global Scope | p. 182 |
Passing Pointers and Arrays to Functions | p. 185 |
Passing a Pointer | p. 185 |
Passing an Array | p. 186 |
Passing Strings | p. 189 |
Returning Pointers | p. 190 |
The main() Function | p. 192 |
Pass Command-Line Arguments to main() | p. 192 |
Passing Numeric Command-Line Arguments | p. 194 |
Function Prototypes | p. 196 |
Headers Contain Prototypes | p. 198 |
Recursion | p. 198 |
The Quicksort | p. 201 |
Module 5 Mastery Check | p. 205 |
A Closer Look at Functions | p. 207 |
Two Approaches to Argument Passing | p. 208 |
How C++ Passes Arguments | p. 208 |
Using a Pointer to Create a Call-by-Reference | p. 209 |
Reference Parameters | p. 211 |
Returning References | p. 216 |
Independent References | p. 219 |
A Few Restrictions When Using References | p. 220 |
Function Overloading | p. 221 |
Automatic Type Conversions and Overloading | p. 225 |
Create Overloaded Output Functions | p. 228 |
Default Function Arguments | p. 234 |
Default Arguments Versus Overloading | p. 235 |
Using Default Arguments Correctly | p. 237 |
Function Overloading and Ambiguity | p. 238 |
Module 6 Mastery Check | p. 241 |
More Data Types and Operators | p. 243 |
The const and volatile Qualifiers | p. 244 |
const | p. 244 |
volatile | p. 246 |
Storage Class Specifiers | p. 247 |
auto | p. 247 |
extern | p. 247 |
static Variables | p. 249 |
register Variables | p. 253 |
Enumerations | p. 256 |
typedef | p. 259 |
Bitwise Operators | p. 260 |
AND, OR, XOR, and NOT | p. 261 |
The Shift Operators | p. 266 |
Create Bitwise Rotation Functions | p. 269 |
The ? Operator | p. 274 |
The Comma Operator | p. 275 |
Multiple Assignments | p. 277 |
Compound Assignment | p. 277 |
Using sizeof | p. 278 |
Precedence Summary | p. 279 |
Module 7 Mastery Check | p. 280 |
Classes and Objects | p. 283 |
Class Fundamentals | p. 284 |
The General Form of a Class | p. 284 |
Defining a Class and Creating Objects | p. 285 |
Adding Member Functions to a Class | p. 289 |
Creating a Help Class | p. 292 |
Constructors and Destructors | p. 298 |
Parameterized Constructors | p. 300 |
Adding a Constructor to the Vehicle Class | p. 302 |
An Initialization Alternative | p. 304 |
Inline Functions | p. 305 |
Creating Inline Functions Inside a Class | p. 307 |
Creating a Queue Class | p. 310 |
Arrays of Objects | p. 315 |
Initializing Object Arrays | p. 316 |
Pointers to Objects | p. 318 |
Object References | p. 320 |
Module 8 Mastery Check | p. 320 |
A Closer Look at Classes | p. 323 |
Overloading Constructors | p. 324 |
Assigning Objects | p. 325 |
Passing Objects to Functions | p. 327 |
Constructors, Destructors, and Passing Objects | p. 328 |
Passing Objects by Reference | p. 330 |
A Potential Problem When Passing Objects | p. 332 |
Returning Objects | p. 332 |
Creating and Using a Copy Constructor | p. 335 |
Friend Functions | p. 338 |
Structures and Unions | p. 343 |
Structures | p. 343 |
Unions | p. 345 |
The this Keyword | p. 349 |
Operator Overloading | p. 351 |
Operator Overloading Using Member Functions | p. 352 |
Order Matters | p. 355 |
Using Member Functions to Overload Unary Operators | p. 356 |
Nonmember Operator Functions | p. 361 |
Using a Friend to Overload a Unary Operator | p. 365 |
Operator Overloading Tips and Restrictions | p. 366 |
Creating a Set Class | p. 368 |
Module 9 Mastery Check | p. 378 |
Inheritance, Virtual Functions, and Polymorphism | p. 379 |
Inheritance Fundamentals | p. 380 |
Member Access and Inheritance | p. 383 |
Base Class Access Control | p. 386 |
Using protected Members | p. 388 |
Constructors and Inheritance | p. 391 |
Calling Base Class Constructors | p. 393 |
Extending the Vehicle Class | p. 398 |
Creating a Multilevel Hierarchy | p. 401 |
Inheriting Multiple Base Classes | p. 404 |
When Constructor and Destructor Functions Are Executed | p. 406 |
Pointers to Derived Types | p. 408 |
References to Derived Types | p. 409 |
Virtual Functions and Polymorphism | p. 409 |
Virtual Function Fundamentals | p. 409 |
Virtual Functions Are Inherited | p. 412 |
Why Virtual Functions? | p. 413 |
Applying Virtual Functions | p. 414 |
Pure Virtual Functions and Abstract Classes | p. 419 |
Module 10 Mastery Check | p. 423 |
The C++ I/O System | p. 425 |
Old vs. Modern C++ I/O | p. 426 |
C++ Streams | p. 426 |
The C++ Predefined Streams | p. 427 |
The C++ Stream Classes | p. 428 |
Overloading the I/O Operators | p. 429 |
Creating Inserters | p. 429 |
Using Friend Functions to Overload Inserters | p. 431 |
Overloading Extractors | p. 432 |
Formatted I/O | p. 434 |
Formatting with the ios Member Functions | p. 435 |
Using I/O Manipulators | p. 440 |
Creating Your Own Manipulator Functions | p. 443 |
File I/O | p. 446 |
Opening and Closing a File | p. 446 |
Reading and Writing Text Files | p. 448 |
Unformatted and Binary I/O | p. 450 |
Reading and Writing Blocks of Data | p. 453 |
More I/O Functions | p. 454 |
More Versions of get() | p. 455 |
getline() | p. 456 |
Detecting EOF | p. 456 |
peek() and putback() | p. 456 |
flush() | p. 457 |
A File Comparison Utility | p. 457 |
Random Access | p. 461 |
Checking I/O Status | p. 464 |
Module 11 Mastery Check | p. 465 |
Exceptions, Templates, and Other Advanced Topics | p. 467 |
Exception Handling | p. 468 |
Exception Handling Fundamentals | p. 468 |
Using Multiple catch Statements | p. 473 |
Catching All Exceptions | p. 476 |
Specifying Exceptions Thrown by a Function | p. 477 |
Rethrowing an Exception | p. 478 |
Templates | p. 480 |
Generic Functions | p. 481 |
A Function with Two Generic Types | p. 483 |
Explicitly Overloading a Generic Function | p. 483 |
Generic Classes | p. 485 |
Explicit Class Specializations | p. 488 |
Creating a Generic Queue Class | p. 490 |
Dynamic Allocation | p. 494 |
Initializing Allocated Memory | p. 496 |
Allocating Arrays | p. 497 |
Allocating Objects | p. 498 |
Namespaces | p. 502 |
Namespace Fundamentals | p. 503 |
using | p. 506 |
Unnamed Namespaces | p. 508 |
The std Namespace | p. 509 |
static Class Members | p. 510 |
static Member Variables | p. 510 |
static Member Functions | p. 512 |
Runtime Type Identification (RTTI) | p. 514 |
The Casting Operators | p. 518 |
dynamic_cast | p. 518 |
const_cast | p. 519 |
static_cast | p. 520 |
reinterpret_cast | p. 520 |
What Next? | p. 520 |
Module 12 Mastery Check | p. 521 |
The Preprocessor | p. 523 |
#define | p. 524 |
Function-Like Macros | p. 526 |
#error | p. 528 |
#include | p. 528 |
Conditional Compilation Directives | p. 529 |
#if, #else, #elif, and #endif | p. 529 |
#ifdef and #ifndef | p. 531 |
#undef | p. 532 |
Using defined | p. 532 |
#line | p. 533 |
#pragma | p. 533 |
The # and ## Preprocessor Operators | p. 534 |
Predefined Macro Names | p. 535 |
Working with an Older C++ Compiler | p. 537 |
Two Simple Changes | p. 539 |
Index | p. 541 |
Table of Contents provided by Ingram. All Rights Reserved. |
ISBN: 9780072232158
ISBN-10: 0072232153
Series: Beginner's Guides (McGraw-Hill)
Published: 1st December 2003
Format: Paperback
Language: English
Number of Pages: 576
Audience: General Adult
Publisher: McGraw Hill
Country of Publication: US
Edition Number: 2
Edition Type: Revised
Dimensions (cm): 23.5 x 19 x 3.5
Weight (kg): 1.09
Shipping
Standard Shipping | Express Shipping | |
---|---|---|
Metro postcodes: | $9.99 | $14.95 |
Regional postcodes: | $9.99 | $14.95 |
Rural postcodes: | $9.99 | $14.95 |
How to return your order
At Booktopia, we offer hassle-free returns in accordance with our returns policy. If you wish to return an item, please get in touch with Booktopia Customer Care.
Additional postage charges may be applicable.
Defective items
If there is a problem with any of the items received for your order then the Booktopia Customer Care team is ready to assist you.
For more info please visit our Help Centre.
You Can Find This Book In
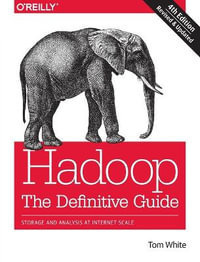
Hadoop : The Definitive Guide
Storage and Analysis at Internet Scale : 4th Edition Revised and Updated
Paperback
RRP $123.50
$48.35
OFF
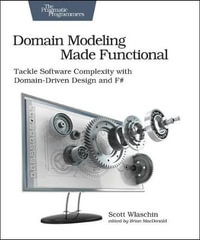
Domain Modeling Made Functional : Pragmatic Programmers
Tackle Software Complexity with Domain-Driven Design and F#
Paperback
RRP $91.25
$44.25
OFF
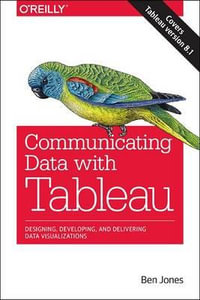
Communicating Data with Tableau
Designing, Developing, and Delivering Data Visualizations : Covers Tableau version 8.1
Paperback
RRP $76.00
$33.75
OFF