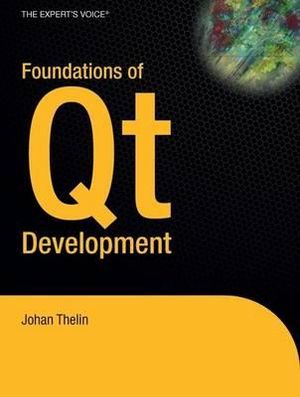
At a Glance
552 Pages
23.5 x 19.05 x 2.85
Paperback
$117.74
or 4 interest-free payments of $29.43 with
orAims to ship in 7 to 10 business days
Qt is a necessary basis for all programmers who want to
develop cross-platform applications on Windows, Mac OS,
Linux, and FreeBSD. A multitude of popular applications
have been written in Qt including Adobe Photoshop
Elements, Google Earth, Perforce Visual Client, and
Skype. Foundations of Qt Development is based on Qt 4.2,
and is aimed at C++ programmers who want to become
proficient using this excellent toolkit to create
graphical applications that can be ported to all major
platforms. The book is focused on teaching you to write
your own code in addition to using existing code. And
common areas of confusion are identified, addressed, and
answered. You'll learn everything you need to know to get
up and running fast.
Foreword | p. xv |
About the Author | p. xvii |
About the Technical Reviewer | p. xix |
Acknowledgments | p. xxi |
Getting to Know Qt | |
The Qt Way of C++ | p. 3 |
Installing a Qt Development Environment | p. 3 |
Installing on Unix Platforms | p. 3 |
Installing on Windows | p. 5 |
Making C++ "Qt-er" | p. 6 |
Inheriting Qt | p. 7 |
Using a Qt String | p. 10 |
Building a Qt Program | p. 11 |
Signals, Slots, and Meta-Objects | p. 13 |
Making the Connection | p. 16 |
Revisiting the Build Process | p. 18 |
Connection to Something New | p. 19 |
Collections and Iterators | p. 21 |
Iterating the QList | p. 21 |
Filling the List | p. 24 |
More Lists | p. 24 |
Special Lists | p. 25 |
Summary | p. 31 |
Rapid Application Development Using Qt | p. 33 |
The Sketch | p. 33 |
Event-Driven Applications | p. 34 |
Using Designer | p. 35 |
From Designer to Code | p. 47 |
The Final Touches | p. 53 |
Summary | p. 54 |
Widgets and Layouts | p. 55 |
Creating Dialogs in Qt | p. 55 |
Size Policies | p. 57 |
Layouts | p. 60 |
Common Widgets | p. 62 |
QPushButton | p. 62 |
QLabel | p. 64 |
QLineEdit | p. 65 |
QCheckBox | p. 66 |
QRadioButton | p. 67 |
QGroupBox | p. 68 |
QListWidget | p. 69 |
QComboBox | p. 71 |
QSpinBox | p. 72 |
QSlider | p. 73 |
QProgressBar | p. 74 |
Common Dialogs | p. 75 |
Files | p. 75 |
Messages | p. 79 |
Even More Dialogs | p. 85 |
Validating User Input | p. 86 |
Validators | p. 87 |
Summary | p. 93 |
The Main Window | p. 95 |
Windows and Documents | p. 95 |
Single Document Interface | p. 96 |
Multiple Document Interface | p. 103 |
Comparing Single and Multiple Document Interfaces | p. 111 |
Application Resources | p. 112 |
Resource File | p. 112 |
Project File | p. 114 |
Application Icon | p. 114 |
Dockable Widgets | p. 115 |
Summary | p. 119 |
The Qt Building Blocks | |
The Model-View Framework | p. 123 |
Showing Data by Using Views | p. 124 |
Providing Headers | p. 127 |
Limiting Editing | p. 127 |
Limiting Selection Behavior | p. 127 |
A Single Column List | p. 128 |
Creating Custom Views | p. 129 |
A Delegate for Drawing | p. 129 |
Custom Editing | p. 132 |
Creating Your Own Views | p. 135 |
Creating Custom Models | p. 140 |
A Read-Only Table Model | p. 141 |
A Tree of Your Own | p. 144 |
Editing the Model | p. 150 |
Sorting and Filtering Models | p. 153 |
Summary | p. 156 |
Creating Widgets | p. 157 |
Composing Widgets | p. 157 |
Changing and Enhancing Widgets | p. 162 |
Catching the Events | p. 164 |
Creating Custom Widgets from Scratch | p. 171 |
Your Widgets and Designer | p. 176 |
Promotion | p. 176 |
Providing a Plugin | p. 177 |
Summary | p. 182 |
Drawing and Printing | p. 183 |
Drawing Widgets | p. 183 |
The Drawing Operations | p. 184 |
Transforming the Reality | p. 200 |
Painting Widgets | p. 204 |
The Graphics View | p. 215 |
Interacting Using a Custom Item | p. 220 |
Printing | p. 228 |
OpenGL | p. 232 |
Summary | p. 232 |
Files, Streams, and XML | p. 235 |
Working with Paths | p. 235 |
Working with Files | p. 238 |
Working with Streams | p. 239 |
XML | p. 243 |
DOM | p. 244 |
Reading XML Files with SAX | p. 248 |
Files and the Main Window | p. 250 |
Summary | p. 255 |
Providing Help | p. 257 |
Creating Tooltips | p. 257 |
Creating HTML-Formatted Tooltips | p. 259 |
Inserting Images into Tooltips | p. 260 |
Applying Multiple Tooltips to a Widget | p. 260 |
Providing What's This Help Tips | p. 263 |
Embedding Links into What's This Help Tips | p. 264 |
Taking Advantage of the Status Bar | p. 267 |
Creating Wizards | p. 269 |
Assisting the User | p. 275 |
Creating the Help Documentation | p. 275 |
Putting It Together | p. 277 |
Summary | p. 278 |
Internationalization and Localization | p. 279 |
Translating an Application | p. 279 |
Extracting the Strings | p. 281 |
Linguist: A Tool for Translating | p. 281 |
Set Up a Translation Object | p. 284 |
Qt Strings | p. 285 |
Dealing with Other Translation Cases | p. 287 |
Find the Missing Strings | p. 291 |
Translating on the Fly | p. 292 |
Other Considerations | p. 295 |
Dealing with Text | p. 295 |
Images | p. 296 |
Numbers | p. 296 |
Dates and Times | p. 298 |
Help | p. 301 |
Summary | p. 301 |
Plugins | p. 303 |
Plugin Basics | p. 303 |
Extending Qt with Plugins | p. 304 |
Creating an ASCII Art Plugin | p. 304 |
Extending Your Application Using Plugins | p. 317 |
Filtering Images | p. 317 |
Merging the Plugin and the Application | p. 323 |
A Factory Interface | p. 326 |
Non-Qt Plugins | p. 329 |
Summary | p. 332 |
Doing Things in Parallel | p. 333 |
Basic Threading | p. 333 |
Building a Simple Threading Application | p. 334 |
Synchronizing Safely | p. 336 |
Protecting Your Data | p. 338 |
Protected Counting | p. 339 |
Locking for Reading and Writing | p. 341 |
Sharing Resources Among Threads | p. 344 |
Getting Stuck | p. 345 |
Producers and Consumers | p. 347 |
Signaling Across the Thread Barrier | p. 352 |
Passing Strings Between Threads | p. 353 |
Sending Your Own Types Between Threads | p. 356 |
Threads, QObjects, and Rules | p. 359 |
Pitfalls when Threading | p. 359 |
The User Interface Thread | p. 360 |
Working with Processes | p. 363 |
Running uic | p. 363 |
The Shell and Directions | p. 368 |
Summary | p. 368 |
Databases | p. 371 |
A Quick Introduction to SQL | p. 371 |
What Is a Database? | p. 371 |
Inserting, Viewing, Modifying, and Deleting Data | p. 372 |
More Tables Mean More Power | p. 375 |
Counting and Calculating | p. 377 |
Qt and Databases | p. 378 |
Making the Connection | p. 378 |
Querying Data | p. 380 |
Establishing Several Connections | p. 382 |
Putting It All Together | p. 382 |
The Structure of the Application | p. 384 |
The User Interface | p. 384 |
The Database Class | p. 392 |
Putting Everything Together | p. 397 |
Model Databases | p. 398 |
The Query Model | p. 399 |
The Table Model | p. 399 |
The Relational Table Model | p. 400 |
Summary | p. 402 |
Networking | p. 403 |
Using the QtNetwork Module | p. 403 |
Working with Client Protocols | p. 403 |
Creating an FTP Client | p. 404 |
Creating an HTTP Client | p. 417 |
Sockets | p. 424 |
Reliability's Role with UDP and TCP | p. 424 |
Servers, Clients, and Peers | p. 425 |
Sending Images Using TCP | p. 425 |
Broadcasting Pictures Using UDP | p. 436 |
Summary | p. 443 |
Building Qt Projects | p. 445 |
QMake | p. 445 |
The QMake Project File | p. 445 |
Working with Different Platforms | p. 450 |
Building Libraries with QMake | p. 453 |
Building Complex Projects with QMake | p. 454 |
The CMake Build System | p. 457 |
Managing a Simple Application with QMake | p. 457 |
Working with Different Platforms | p. 461 |
Building Libraries with CMake | p. 465 |
Managing Complex Projects with CMake | p. 466 |
Summary | p. 469 |
Unit Testing | p. 471 |
Unit Testing and Qt | p. 472 |
The Structure of a Test | p. 472 |
Testing Dates | p. 474 |
Implementing the Tests | p. 475 |
Data-Driven Testing | p. 479 |
Testing Widgets | p. 483 |
Testing a Spin Box | p. 483 |
Driving Widgets with Data | p. 487 |
Testing Signals | p. 490 |
Testing for Real | p. 491 |
The Interface | p. 492 |
The Tests | p. 492 |
Handling Deviations | p. 497 |
Summary | p. 497 |
Appendixes | |
Third-Party Tools | p. 501 |
Qt Widgets for Technical Applications: Qwt | p. 502 |
wwWidgets | p. 503 |
QDevelop | p. 504 |
Edyuk | p. 505 |
Containers, Types, and Macros | p. 507 |
Containers | p. 507 |
Sequences | p. 507 |
Specialized Containers | p. 508 |
Associative Containers | p. 509 |
Types | p. 509 |
Types by Size | p. 509 |
The Variant Type | p. 510 |
Macros and Functions | p. 511 |
Treating Values | p. 511 |
Random Values | p. 511 |
Iterating | p. 512 |
Index | p. 513 |
Table of Contents provided by Ingram. All Rights Reserved. |
ISBN: 9781590598313
ISBN-10: 1590598318
Series: Expert's Voice in Open Source
Published: 1st August 2007
Format: Paperback
Language: English
Number of Pages: 552
Audience: General Adult
Publisher: Springer Nature B.V.
Country of Publication: US
Dimensions (cm): 23.5 x 19.05 x 2.85
Weight (kg): 0.95
Shipping
Standard Shipping | Express Shipping | |
---|---|---|
Metro postcodes: | $9.99 | $14.95 |
Regional postcodes: | $9.99 | $14.95 |
Rural postcodes: | $9.99 | $14.95 |
How to return your order
At Booktopia, we offer hassle-free returns in accordance with our returns policy. If you wish to return an item, please get in touch with Booktopia Customer Care.
Additional postage charges may be applicable.
Defective items
If there is a problem with any of the items received for your order then the Booktopia Customer Care team is ready to assist you.
For more info please visit our Help Centre.
You Can Find This Book In
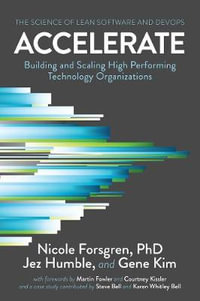
Accelerate
The Science of Lean Software and DevOps: Building and Scaling High Performing Technology Organizations
Paperback
RRP $44.99
$38.90
OFF
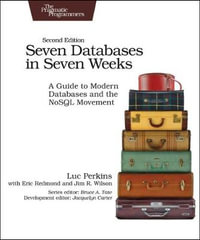
Seven Databases in Seven Weeks : Pragmatic Programmers
A Guide to Modern Databases and the NoSQL Movement : 2nd Edition
Paperback
RRP $91.25
$39.90
OFF
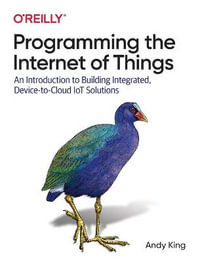
Programming the Internet of Things
An Introduction to Building Integrated, Device-to-Cloud IoT Solutions
Paperback
RRP $152.00
$66.25
OFF