
At a Glance
448 Pages
23.17 x 18.75 x 2.18
Paperback
$64.25
or 4 interest-free payments of $16.06 with
orAims to ship in 10 to 15 business days
When will this arrive by?
Enter delivery postcode to estimate
This friendly, solutions-oriented guide is filled with step-by-step examples that illustrate how to write basic to advanced JavaScript applications, as well as modify existing scripts to suit individual needs. Each chapter begins with the specific how-to topics that will be covered. Within the chapter, each topic is accompanied by a solid, easy-to-follow walkthrough of the process.
Acknowledgments | p. xv |
Introduction | p. xvii |
Learn JavaScript Basics | |
Prepare to Program in JavaScript | p. 3 |
Learn the History of JavaScript | p. 4 |
The Origin of JavaScript | p. 5 |
JavaScript Makes Its Way into Internet Explorer | p. 5 |
JavaScript Becomes an Official Standard | p. 5 |
Where JavaScript Is Today | p. 6 |
Choose a Development Environment | p. 7 |
Develop JavaScript-Enabled Web Pages | p. 7 |
Create Server-Based Web Applications | p. 8 |
Use JavaScript in a DOS or Windows Environment | p. 9 |
JavaScript Development Tools | p. 9 |
Learn What JavaScript Can and Cannot Do | p. 13 |
Use JavaScript as a Client-Side Language | p. 14 |
Use JavaScript as a Server-Side Language | p. 14 |
Decide Which Version of JavaScript to Use | p. 15 |
Test JavaScript Programs Using HTML | p. 16 |
Create a JavaScript Template | p. 16 |
Understand the JavaScript Template | p. 18 |
Communicate with the User | p. 19 |
Display an Alert Message | p. 20 |
Write Text to the Browser Window | p. 21 |
Learn More about Topics Discussed in this Chapter | p. 22 |
Learn JavaScript Fundamentals | p. 25 |
Understand Basic Terminology | p. 26 |
Store Data in Variables | p. 26 |
Define Variables | p. 27 |
Define Constants | p. 28 |
Understand Program Flow | p. 29 |
Control Program Flow with Statements | p. 30 |
Execute Code Conditionally | p. 30 |
Repeat Statements Using Loops | p. 34 |
Comment Your Code | p. 43 |
Set a Default Object | p. 44 |
Handle Errors | p. 46 |
Understand the Basics of Expressions | p. 49 |
Use Operators to Create Complex Expressions | p. 50 |
Organize Your Code into Functions | p. 51 |
Define Functions | p. 52 |
Accept Parameters | p. 54 |
Understand Variable Scope | p. 56 |
Return Values | p. 58 |
Use the Improvements in JavaScript 2.0 to Create More Powerful Functions | p. 59 |
Use Named Optional Parameters | p. 60 |
Accept Any Number of Parameters | p. 61 |
Use Built-in JavaScript Classes | p. 63 |
Learn about Objects in JavaScript | p. 64 |
Write Unstructured Programs | p. 65 |
Organize Code into Procedures | p. 65 |
Separate a Program into Modules | p. 66 |
Use the Object-Oriented Approach | p. 66 |
Turn Properties and Functions into a Class | p. 68 |
JavaScript's Built-in Classes and Data Types | p. 69 |
Instantiate an Object with the new Operator | p. 72 |
Access an Object with the Operator | p. 73 |
Access an Object with the [] Operator | p. 73 |
Create a String Object in JavaScript | p. 73 |
Create a String Object Using a String Literal | p. 74 |
Create a String Object Using the String Data Type | p. 76 |
Use the String Object's Built-in Functionality | p. 76 |
Perform Mathematical Functions | p. 78 |
Apply JavaScript's Date-Handling Functions | p. 79 |
Convert Strings into Numbers | p. 82 |
Use the parseInt and parseFloat Functions | p. 82 |
Prepare Text Before Sending to Web Server | p. 84 |
Use the escape and unescape Functions | p. 85 |
Decide When to Use Regular Expressions | p. 86 |
Understand the Basics of Regular Expressions | p. 87 |
Create Patterns with a RegExp Object | p. 89 |
Understand JavaScript 2.0's Powerful New Data Types | p. 91 |
Use the Boolean, Integer, and Number Data Types | p. 91 |
Use the char Data Type | p. 91 |
Use the Object Data Type | p. 92 |
Understand Special Data Types | p. 92 |
Organize Data into Arrays | p. 95 |
Create an Array Object | p. 97 |
Create an Empty Array | p. 97 |
Specify an Initial Array Length | p. 99 |
Create and Initialize an Array in One Line of Code | p. 100 |
Use Array Literals | p. 100 |
Call the Properties and Methods of the Array Object | p. 101 |
Set and Retrieve Values in an Array | p. 104 |
Use Multidimensional Arrays | p. 107 |
Use JavaScript 2.0's Enhanced Arrays | p. 111 |
The StaticArray Class | p. 112 |
The DynamicArray Class | p. 114 |
The ConstArray Class | p. 114 |
Create Your Own JavaScript Classes | p. 117 |
Learn about Classes in JavaScript | p. 118 |
Create Objects in JavaScript 1.x | p. 118 |
Call a Constructor Function | p. 119 |
Use an Object Literal | p. 122 |
Extend an Existing Class | p. 123 |
Extend an Existing Object | p. 125 |
Create Objects in JavaScript 2.0 | p. 126 |
Define Your Own Classes | p. 127 |
Organize Classes Using Inheritance | p. 130 |
Choose Between Static and Instance Members | p. 135 |
Make Class Members Public or Private | p. 136 |
Build JavaScript-Enabled Web Sites | |
Embed JavaScript in a Web Page | p. 141 |
Understand Basic HTML Structure | p. 142 |
Build an HTML Document | p. 145 |
Indicate the Document Type with [left angle bracket]!DOCTYPE[right angle bracket] | p. 146 |
Add a Title and Define Document Keywords | p. 147 |
Format Text with HTML Elements | p. 148 |
Format Text with Style Sheets | p. 150 |
Use [left angle bracket]script[right angle bracket] to Add JavaScript to a Web Page | p. 153 |
Use [left angle bracket]noscript[right angle bracket] for Browsers That Don't Support Scripting | p. 154 |
Load an External JavaScript File | p. 157 |
Call JavaScript Using Hyperlinks | p. 158 |
Learn More about the Topics in this Chapter | p. 159 |
Create Scripts That Work in Every Browser | p. 161 |
Understand Browser Differences | p. 162 |
What Kind of Errors Can Occur? | p. 163 |
Detect What Type of Browser the User Is Running | p. 164 |
Query the Document Model | p. 169 |
Stick to Web Standards | p. 170 |
Write Cross-Browser Code | p. 171 |
Manipulate Web Forms | p. 179 |
Understand HTML Forms | p. 180 |
Request User Input Using an HTML Form | p. 181 |
Process Form Input with Client-Side JavaScript | p. 182 |
Process Form Input on a Web Server | p. 183 |
Insert an HTML Form into a Web Page | p. 184 |
Retrieve and Set Form Control Values in JavaScript | p. 200 |
Access Form Values Using the forms Array | p. 200 |
Access Form Values Using the elements Array | p. 202 |
Access Form Values Using getElementById() | p. 204 |
Access Form Values Using getElementsByName() | p. 204 |
Access Form Values Using getElementsByTagName() | p. 205 |
Handle Browser Events | p. 207 |
Write JavaScript Event Handlers | p. 208 |
Handle User Interface Events | p. 209 |
Handle Mouse Events | p. 211 |
Handle Key Events | p. 212 |
Handle HTML Events | p. 215 |
Handle Events Using the Event Property | p. 218 |
Trigger Events in JavaScript | p. 219 |
Call the Method Associated with an Event | p. 219 |
Use the fireEvent Method | p. 220 |
Overcome Browser Incompatibility | p. 220 |
Communicate Between Browser Frames | p. 223 |
Learn the Basics of HTML Frames | p. 224 |
Create a Frameset in HTML | p. 225 |
Define and Name Frames in a Frameset | p. 233 |
Call JavaScript Functions from Other Frames | p. 235 |
Access Another Frame Using JavaScript | p. 236 |
Call a JavaScript Function Located in Another Frame | p. 237 |
Handle Synchronization Between Frames | p. 239 |
Interact with the Web Browser | p. 243 |
Learn the Basics of the Document Object Model | p. 245 |
Manipulate the Contents of a Web Page | p. 248 |
Dynamically Modify the Contents of a Web Page | p. 249 |
Change the Items in a Drop-Down List Box | p. 251 |
Examine the Entire Browser Window | p. 252 |
Retrieve Properties of the Web Browser Software | p. 254 |
Examine the Operating System's Display Settings | p. 256 |
Access the Web Browser History List | p. 257 |
Send the Browser to a New Location | p. 258 |
Perform Simple Animation | p. 259 |
Learn the Basics of Cascading Style Sheets | p. 260 |
Assign Style to Web Pages Using HTML Elements | p. 261 |
Assign Style to Web Pages Using Style Sheets | p. 264 |
Use Basic Style Attributes | p. 268 |
Position Elements on a Web Page | p. 268 |
Modify Styles Using JavaScript | p. 270 |
Understand Cross-Platform Issues | p. 272 |
Perform Basic Animation Using JavaScript | p. 274 |
Dynamically Load Images | p. 274 |
Make Content Move Around the Screen | p. 276 |
Take JavaScript to the Next Level | |
Debug JavaScript Programs | p. 281 |
Understand the Possible Causes of Errors | p. 282 |
Find the Source of an Error Message | p. 284 |
Interpret Error Messages | p. 285 |
Use a JavaScript Validator | p. 287 |
Add Debugging Code to Your Programs | p. 287 |
Use the JavaScript Console | p. 290 |
Use a JavaScript Debugger | p. 291 |
Make Your Program Errorproof | p. 295 |
Learn the Basics of Exceptions | p. 296 |
Catch Exceptions Using the try and catch Statements | p. 297 |
Understand Exception Bubbling | p. 299 |
Use the IE Error Object | p. 301 |
Use Netscape-Only catch Clauses | p. 302 |
Use Nonstandard finally Clauses | p. 302 |
Create Exceptions Using the throw Statement | p. 304 |
Design Programs That Are Easy to Debug from the Start | p. 306 |
Avoid Unstructured Programming | p. 307 |
Break Code into Manageable Chunks | p. 307 |
Reuse Code Using Classes and Objects | p. 308 |
Test Your JavaScript Code Thoroughly | p. 309 |
Create a Testing Harness | p. 309 |
Force Errors to Test Error-Handling Code | p. 311 |
Try Your Program in Many Different Environments | p. 312 |
Use JavaScript to Manage Browser Plug-Ins | p. 313 |
Insert Scriptable Objects into HTML Web Pages | p. 315 |
Include Sun Java Applets | p. 315 |
Connect to Java Applets Using JavaScript | p. 319 |
Embed Movies and Music in Web Pages | p. 321 |
Connect to Music and Media Objects Using JavaScript | p. 322 |
Use the Microsoft Calendar Control in Your Web Pages | p. 324 |
HTML 4.01 Tags | p. 327 |
JavaScript Quick Reference | p. 331 |
Index | p. 335 |
Table of Contents provided by Rittenhouse. All Rights Reserved. |
ISBN: 9780072228878
ISBN-10: 0072228873
Series: How to Do Everything
Published: 17th March 2003
Format: Paperback
Language: English
Number of Pages: 448
Audience: Professional and Scholarly
Publisher: OSBORNE
Country of Publication: US
Edition Number: 1
Dimensions (cm): 23.17 x 18.75 x 2.18
Weight (kg): 0.59
Shipping
Standard Shipping | Express Shipping | |
---|---|---|
Metro postcodes: | $9.99 | $14.95 |
Regional postcodes: | $9.99 | $14.95 |
Rural postcodes: | $9.99 | $14.95 |
How to return your order
At Booktopia, we offer hassle-free returns in accordance with our returns policy. If you wish to return an item, please get in touch with Booktopia Customer Care.
Additional postage charges may be applicable.
Defective items
If there is a problem with any of the items received for your order then the Booktopia Customer Care team is ready to assist you.
For more info please visit our Help Centre.
You Can Find This Book In
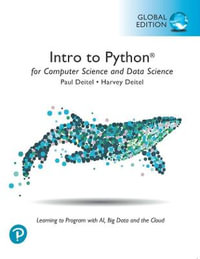
Intro to Python for Computer Science and Data Science
Learning to Program with AI, Big Data and The Cloud, Global Edition
Paperback
RRP $153.95
$123.25
OFF
![Introduction to Java Programming and Data Structures, Comprehensive Version [Global Edition] : 12th Edition - Y. Liang](https://www.booktopia.com.au/covers/200/9781292402079/3378/introduction-to-java-programming-and-data-structures-comprehensive-version-global-edition-.jpg)
Introduction to Java Programming and Data Structures, Comprehensive Version [Global Edition]
12th Edition
Paperback
RRP $134.95
$109.25
OFF
This product is categorised by
- Non-FictionComputing & I.T.Computer Programming & Software DevelopmentObject-Oriented Programming or OOP
- Non-FictionComputing & I.T.Computer Programming & Software DevelopmentWeb Programming
- Non-FictionComputing & I.T.Information Technology General Issue
- Non-FictionComputing & I.T.Operating SystemsApple Operating Systems
- Non-FictionComputing & I.T.Digital Lifestyle & Online World: Consumer & User GuidesInternet Guides & Online Services